Section 9.8 Worked Example: Parameter Passing
Subsection 9.8.1
Problem: Evaluate the following code - what is the output?
public class Parms {
public static void primMethod (int beta, Person mm, Person mm2) {
System.out.println("Inside method primMethod:");
System.out.println("beta is " + beta + " mm is " + mm + " mm2 is " + mm2);
// change values
beta = -22;
mm.setName("Daisy Duck");
mm.setId(932);
mm2 = new Person("Pluto", 87);
System.out.println("At end of method primMethod:");
System.out.println("beta is " + beta + " mm is " + mm + " mm2 is " + mm2);
}
public static void main(String[] args) {
int alpha = 99;
Person mickey = new Person("Mickey Mouse", 111);
Person dupe = mickey;
System.out.println("Before method call:");
System.out.println("alpha is " + alpha + " mickey is " + mickey + " dupe is " + dupe);
primMethod(alpha, mickey, dupe);
System.out.println("After method call:");
System.out.println("alpha is " + alpha + " mickey is " + mickey + " dupe is " + dupe);
}
}
This code also makes use of a Person class, which is defined here:
public class Person {
private String name; // name of person
private int id; // person's id
// overloaded constructor
public Person(String name, int id) {
setName(name);
setId(id);
}
// default constructor
public Person() {
}
// Accessors and Mutators
public String getName() {
return name;
}
public void setName(String name) {
if (name.length() != 0) // name must not be null
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
// toString to allow conversion to String
public String toString() {
return "Person{" + "name='" + name + '\'' + ", id=" + id + '}';
}
// determines if two Person instances are the same
// true if both the name (regardless of case) and id are the same
public boolean equals(Person person) {
if (getId() != person.getId()) return false;
if (getName().equalsIgnoreCase(person.getName()))
return true;
return false;
}
}
Subsection 9.8.2 Evaluate code
Let’s look at exactly what this code is doing.
int alpha = 99;
Person mickey = new Person("Mickey Mouse", 111);
Person dupe = mickey;
The first three lines are just declaring and initializing some variables. Once these statements have executed, here is a memory representation:
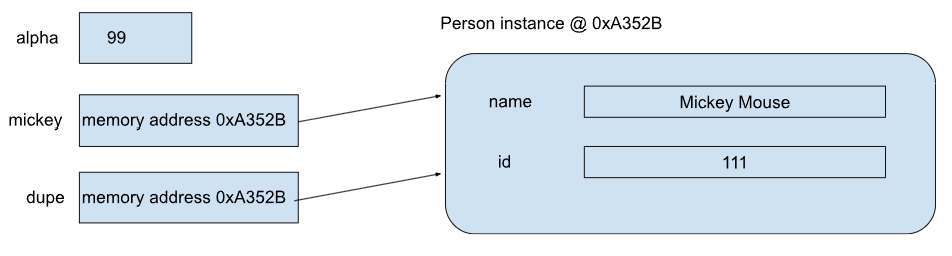
Notice that the object references
mickey
and dupe
both refer to the same Person
instance.The next lines are prints:
System.out.println("Before method call:");
System.out.println("alpha is " + alpha + " mickey is " + mickey + " dupe is " + dupe);
Which will produce the output:
Before method call:
alpha is 99 mickey is Person{name='Mickey Mouse', id=111} dupe is Person{name='Mickey Mouse', id=111}
Because
mickey
and dupe
are referring to the same Person
instance, they print the same values.The next line
primMethod(alpha, mickey, dupe);
is calling the method
primMethod
and passing the arguments of alpha
, mickey
, and dupe
. Here is a memory representation once primMethod
has been called: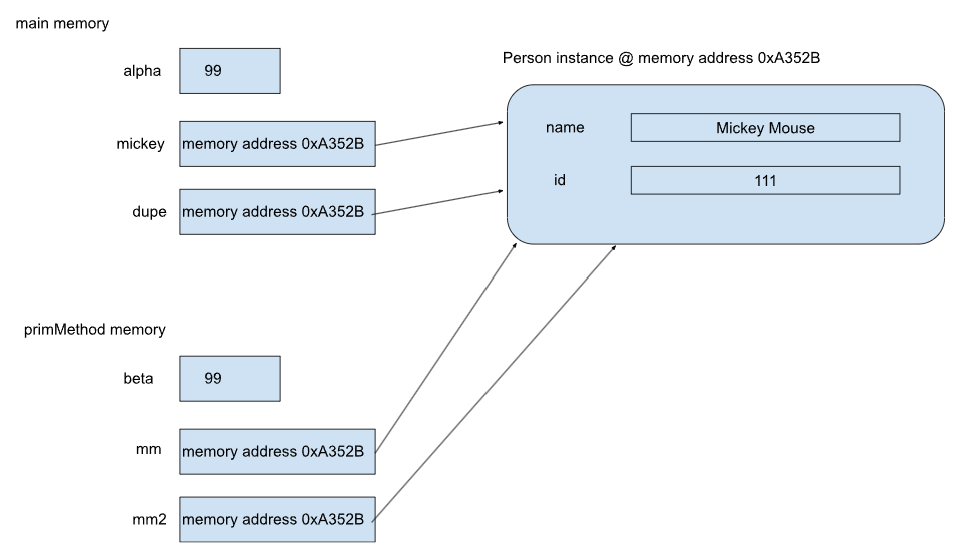
In Java, all arguments are pass-by-value, meaning that a copy of the value is made. So the variable
beta
has a copy of alpha
’s value, mm
has a copy of mickey
’s value, and mm2
has a copy of dupe
’s value. However, because mm
and mm2
are object references, they are still referencing the same Person
instance that was createdWe are now inside the
primMethod
, where the first two lines are printing values of the parameters:System.out.println("Inside method primMethod:");
System.out.println("beta is " + beta + " mm is " + mm + " mm2 is " + mm2);
They will generate this output:
Inside method primMethod:
beta is 99 mm is Person{name='Mickey Mouse', id=111} mm2 is Person{name='Mickey Mouse', id=111}
Next is code that changes the values:
beta = -22;
mm.setName("Daisy Duck");
mm.setId(932);
mm2 = new Person("Pluto", 87);
Here is a memory representation:
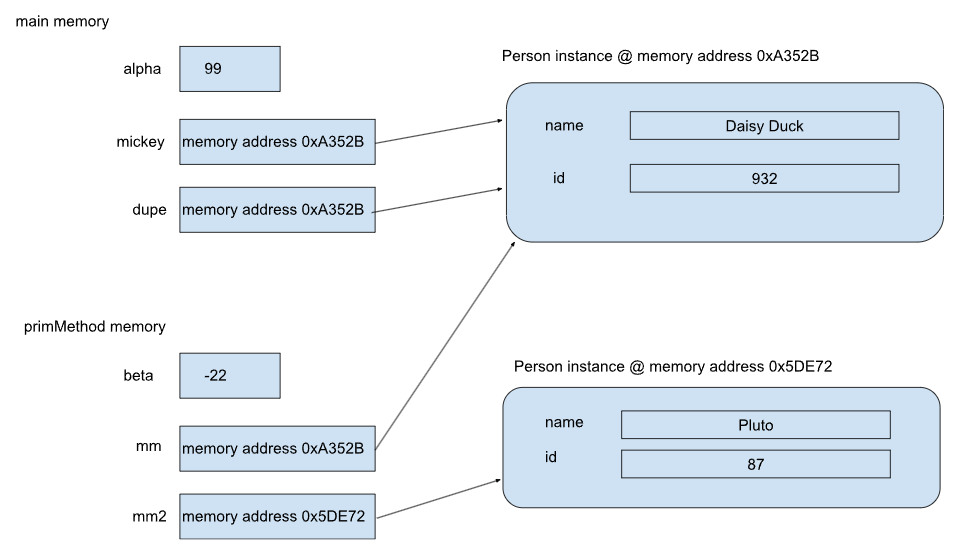
- The value of
beta
has been changed due to the assignment statement. - The contents of the
Person
instance referenced bymm
(andmickey
anddupe
) have been updated. - The
mm2
object reference now refers to a completely newPerson
instance (at a different memory location).
So the final statements inside
primMethod
System.out.println("At end of method primMethod:");
System.out.println("beta is " + beta + " mm is " + mm + " mm2 is " + mm2);
will generate this output:
At end of method primMethod:
beta is -22 mm is Person{name='Daisy Duck', id=932} mm2 is Person{name='Pluto', id=87}
We are now back in the
main()
method immediately after the call to primMethod
. Look at the previous memory representation. Has alpha
’s value changed? What about mickey
’s value or dupe
’s value?Answer.
No, none of the values of those variables have changed, because Java simply makes a copy of the variable values.
However, the object that
mickey
and dupe
refer to has had its content updated by primMethod
.So the final print statements in
main()
System.out.println("After method call:");
System.out.println("alpha is " + alpha + " mickey is " + mickey + " dupe is " + dupe);
Will generate this output:
After method call:
alpha is 99 mickey is Person{name='Daisy Duck', id=932} dupe is Person{name='Daisy Duck', id=932}
Where
alpha
has the same value as before the method call and mickey
and dupe
still refer to the same Person
instance, however the contents of that instance have been updated.Subsection 9.8.3
Answer.
Before method call:
alpha is 99 mickey is Person{name='Mickey Mouse', id=111} dupe is Person{name='Mickey Mouse', id=111}
Inside method primMethod:
beta is 99 mm is Person{name='Mickey Mouse', id=111} mm2 is Person{name='Mickey Mouse', id=111}
At end of method primMethod:
beta is -22 mm is Person{name='Daisy Duck', id=932} mm2 is Person{name='Pluto', id=87}
After method call:
alpha is 99 mickey is Person{name='Daisy Duck', id=932} dupe is Person{name='Daisy Duck', id=932}
You have attempted of activities on this page.