Section 11.15 Worked Example: Arrays - Find Value
Subgoals for Evaluating Arrays.
-
Declaring and initialization of array
- Set up a one dimensional table (i.e., one row) with 0 to (size - 1) elements
- Upon instantiation of an array object, all elements contain default value for datatype stored in array OR values from the initializer list
- Determine access or change of element, or action on entire array object, and update slots as needed (remembering assignment subgoals)
-
Accessing array element
- Evaluate expression within [] which will be the index for element to be accessed
arrayName[index]
returns value stored at that index- index must be between 0 and
arrayName.length
- 1, inclusive otherwiseIndexOutOfBounds
exception occurs
-
Changing value of an array element
- Evaluate expression within [] which will be the index for element to be accessed
arrayName[index]
will now contain the value on the RHS of assignment statement- (remember the assignment subgoals for verifying data types and evaluating expressions)
- (remember rules for index values)
-
Whole array actions
- Pass as argument - a copy of the reference to the instantiated array is passed to the method. This means that any changes made to the array elements inside the method are persistent. The one exception to this is if you assign the argument to reference a different array in memory.
- Assignment - changes the reference to point to the array on the RHS of the assignment operator.
Subsection 11.15.1
Problem: Assume that the integer array
alpha
has been properly declared and initialized with non-default values, and that the variable target
is an int. What does this code accomplish? How would you describe the value in loc
?int loc = -1;
boolean found = false;
for (int i = 0; i < alpha.length && !found; i++) {
if (alpha[i] == target) {
loc = i;
found = true;
}
}
Subsection 11.15.2 SG1: Declaring and initialization of array
There is no explicit declaration or initialization of an array within the code. However, within the code there are [ ], so we know we are accessing an array.

- alpha is an array of ints and has values, but we don’t know what those values are
- however, we can still diagram a representation of this array
- notice that the largest index is size - 1
Subsection 11.15.3 SG2: Determine access or action
Within the loop, we are accessing array elements.
Subsection 11.15.4 Evaluating code
int loc = -1;
boolean found = false;
The first two lines are declaring an integer variable (
loc
) and assigning it a value of -1. This variable will represent the "location" of the target variable, if found. A second variable, found
, is declared and given an initial value of false. This variable represents whether or not we have located the target within the array.for (int i = 0; i < alpha.length && !found; i++) {
if (alpha[i] == target) {
loc = i;
found = true;
}
}
- This loop has index
i
go from 0 tosize
- 1 (<length
) by increments of 1. - Then the value at
alpha[i]
is compared to the int value oftarget
. - If the value at
alpha[i]
is equal totarget
, then the valuei
is copied intoloc
. - All indexes into the array are valid, and all assignments are valid.
Let us trace with a sample array and assume the value of
target
is 15.
The first statement,
int loc = -1;
gives loc
a value that is not a valid index for any array, and we have not yet found the target within the array.Then a for-loop is used to traverse the array and compare each element to
target
. The chart below uses one line to represent the memory and comparisons during each iteration of the loop, starting when i
has a value of zero.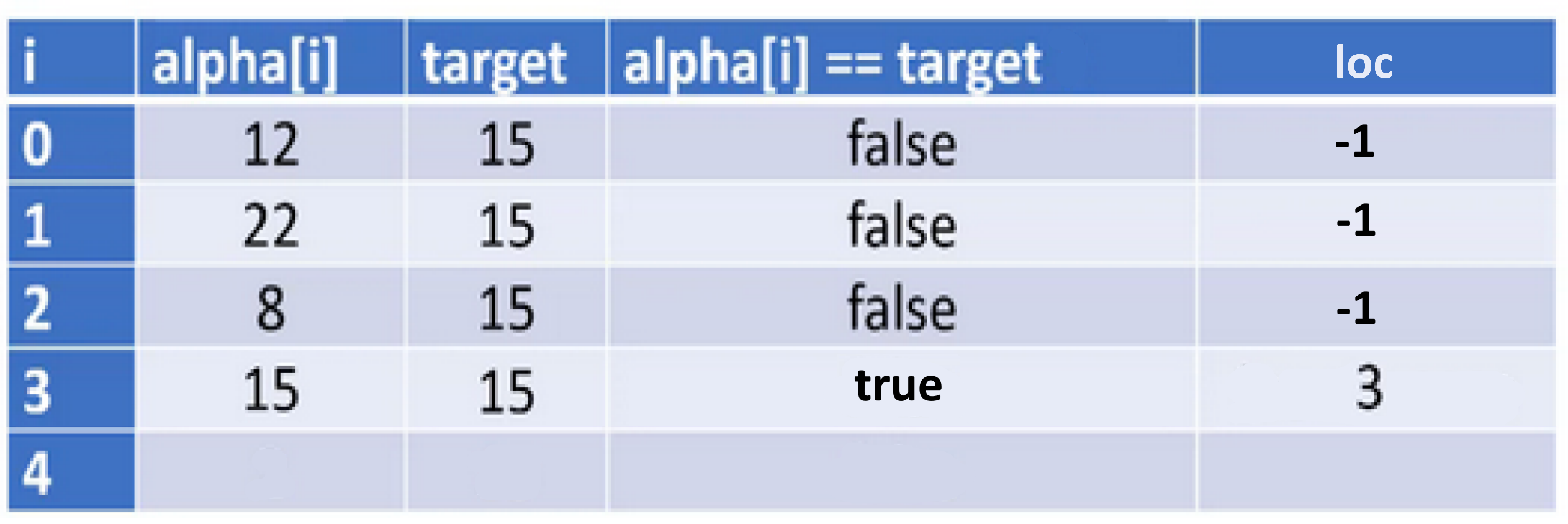
When we find the target value in the array, we store the index of where the value was found into the variable
loc
.When
i
is 4, the for loop continuation condition is evaluated:i < alpha.length && !found;
and
i
(value of 4) is less than alpha.length
(which is 5 in this case) however the variable found
is now true, so !found
is false. true && false
is false, so the loop body is not executed.Some questions to consider:
- What would happen if the
target
value is not in the array?Answer.
Then the selection statement is never true, andloc
is never changed from its initial value of -1. - Why is -1 a good initial value for
loc
?Answer.
It is not a valid index for any array. You or another programmer using this algorithm could check the value ofloc
to make a decision (selection!) for how the program will behave when thetarget
value is found or not found at a valid array index. - What would happen if there were 2 occurrences of the target value in the array?
Answer.
The loop does not end when thetarget
value is found, so no additional occurrences would be evaluated. If the ( && !found) were not included in the code, then any additional occurences of target being found in the array would overwrite the value ofloc
with the last occurence.
What does this code accomplish?
Answer.
loc
contains the index of the first occurrence of target
in the array alpha
or -1 if target
is not in the array.Subsection 11.15.5 Practice Pages
You have attempted of activities on this page.