Checkpoint 10.5.1.
- font color; font size; x,y coordinate; text
- Incorrect. Consult the syntax part of the documentation for the answer.
- x,y coordinate; font size; font color; text
- Incorrect, check the documentation.
- font size; text; x,y coordinate; font color
- Incorrect, the documentation can help you find the answer.
- text; x,y coordinate; font size; font color
- Correct!
What is the correct order of required parameters (arguments) for the function, canvas.draw_text()?
The following image shows part of the documentation associated with this function. Review the complete documentation to find the answer:
https://py3.codeskulptor.org/docs.html#draw_text
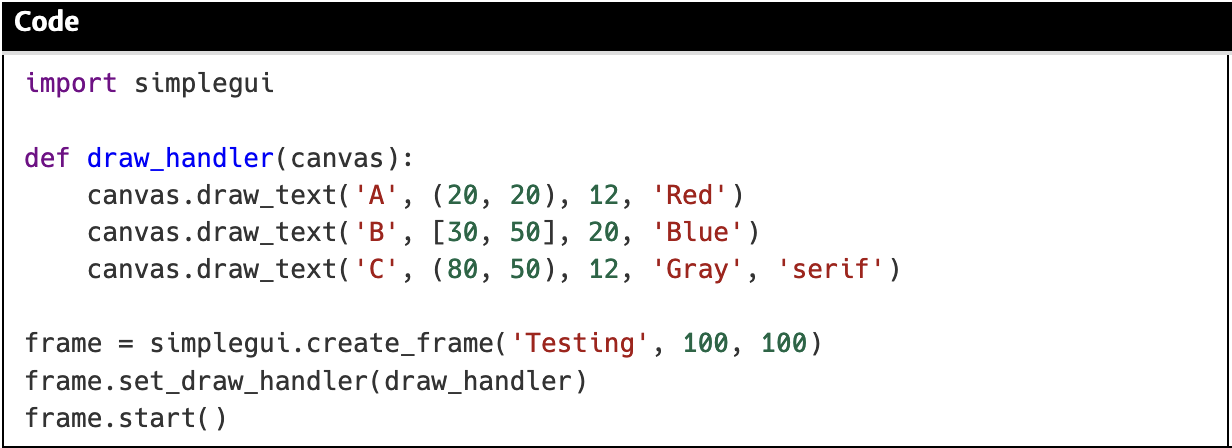