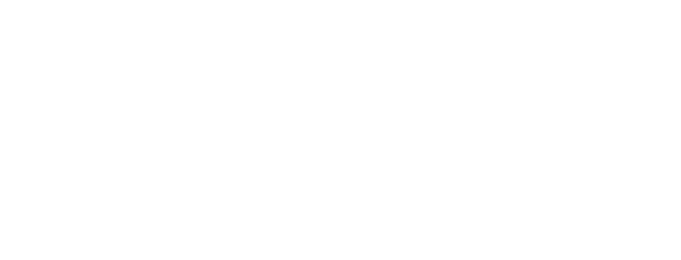
5.6. Quiz App Projects Loops with Lists¶
Time Estimate: 90 minutes
5.6.1. Introduction and Goals¶
In this lesson, you will complete several small programming projects that add enhancements to the Quiz App. You are encouraged to discuss your ideas for how to solve these problems with the instructor and with your partner and other students. Hints and suggestions are provided. | |
Learning Objectives: I will learn to
Language Objectives: I will be able to
|
5.6.2. Learning Activities¶
Loops with Lists:
Here is a quick review of comparing AP pseudocode and App Inventor blocks for loops with list:
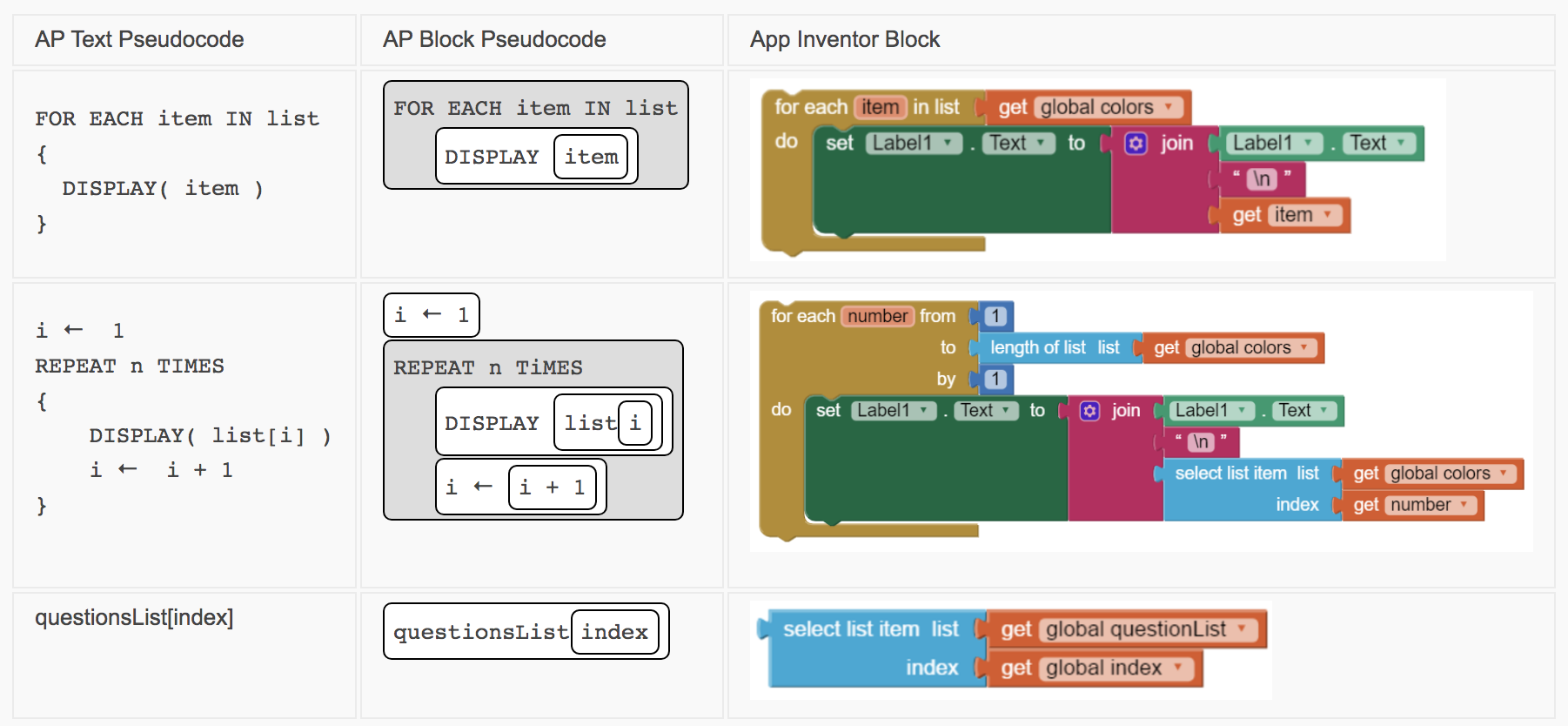
- Accessing an element by index: list[i] where i is an index from 1 to the length of the list.
- Saving an element of a list into a variable like x: x ← list[i]
- Assigning a value to an element of a list:
- list[i] ← x assigns the value of x to list[i].
- list[i] ← list[j] assigns the value of list[j] to list[i].
- INSERT(list, i, value) : inserts value into the list at index i, moving down all other items at and after i in the list.
- APPEND(list, value): adds value to the end of the list.
- REMOVE(list, i): removes the item at index i and moves up all items after the ith item.
- LENGTH(list): evaluates to the number of elements currently in the given list.
Programming and Creative Projects
For this lesson you can start up App Inventor and open the project you created in the previous lesson. After opening your Quiz project, rename it QuizProjects2, for Quiz Version 2 -- or something similar to that. Then complete the programming exercises described below.
- If/else Scoring Algorithm: Modify your app to keep score of how many questions are answered correctly or incorrectly. Be sure to restrict it so that the quiz taker can only receive credit for answering each question once (i.e., if there are three questions, the quiz taker can only be credited with three correct answers). Use this short handout to guide you with this project.
- Loop Algorithm for Searching: Add a keyword search capability to your app. For example, if the user types in NASA and clicks on the search button, you should find the question or answer with the word NASA in it and show that question. This will be a linear search through the parallel question and answer lists using a loop. Use this short handout to guide you with this project.
- Your Own Quiz App: Use the Quiz App as a template to create a quiz on a topic of your own choosing. Besides changing the questions, answers, and pictures, add at least one enhancement to the app. Be creative!
5.6.3. Summary¶
In this lesson, you learned how to:
5.6.4. Self-Check¶
Vocabulary
Here is a table of the technical terms we've introduced in this lesson. Hover over the terms to review the definitions.
insert
append length |
Check Your Understanding
You can practice more algorithms with loops and lists below. It is useful to know standard algorithms that use loops like searching for an item in a list, finding the minimum or maximum value in a list, computing the sum or average of a list of values, etc. Using existing algorithms as building blocks for constructing new algorithms has benefits such as reducing development time, reducing testing, and simplifying the identification of errors.
- Displays 0 which is the minimum (lowest) value in the list.
- No, 0 is not greater than 1.
- Displays 1 which is the first item in the list.
- No, 1 is replaced the third time through the loop.
- Displays -1 which is the value of x.
- No, the items in the list replace x's -1 value.
- Displays 4 which is the maximum (largest) value in the list.
- That's correct!
Q-3:
What does the following code do?
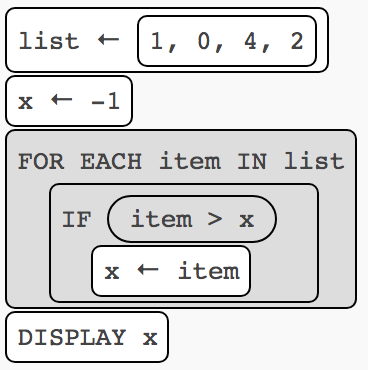
Q-4:
Which code below could be placed in the following loop to print out the item in a list that has the lowest (minimum) value?
list ← [1, 0, 4, 2] x ← 99 FOR EACH item IN list { <MISSING CODE> } DISPLAY(x)
x ← item
x ← item
x ← item
x ← item
Q-5:
What are the values in the list after executing the following code:
list ← [ 0, 3, 5 ] APPEND( list, 4 ) INSERT( list, 2, 1 ) REMOVE( list, 1 )
Sample AP CSP Exam Questions
Q-6:
A summer camp offers a morning session and an afternoon session.
The list morningList contains the names of all children attending the morning session, and the list afternoonList contains the names of all children attending the afternoon session.
Only children who attend both sessions eat lunch at the camp. The camp director wants to create lunchList, which will contain the names of children attending both sessions.
The following code segment is intended to create lunchList, which is initially empty. It uses the procedure IsFound (list, name), which returns true if name is found in list and returns false otherwise.
FOR EACH child IN morningList { <MISSING CODE> }
Which of the following could replace <MISSING CODE> so that the code segment works as intended?
IF (IsFound (afternoonList, child))
{
APPEND (lunchList, child)
}
IF (IsFound (lunchList, child))
{
APPEND (afternoonList, child)
}
IF (IsFound (morningList, child))
{
APPEND (lunchList, child)
}
IF ((IsFound (morningList, child)) OR
(IsFound (afternoonList, child)))
{
APPEND (lunchList, child)
}
Q-7:
A teacher uses the following program to adjust student grades on an assignment by adding 5 points to each student’s original grade. However, if adding 5 points to a student’s original grade causes the grade to exceed 100 points, the student will receive the maximum possible score of 100 points. The students’ original grades are stored in the list gradeList, which is indexed from 1 to n.
i ← 1 REPEAT n TIMES { <MISSING CODE> i ← i + 1 }
The teacher has the following procedures available.

Which of the following code segments can replace <MISSING CODE> so that the program works as intended?
Select two answers.
gradeList[i] ← min (gradeList[i] + 5, 100)
gradeList[i] ← max (gradeList[i] + 5, 100)
gradeList[i] ← gradeList[i] + 5
IF (gradeList[i] > 100)
{
	gradeList[i] ← gradeList[i] - 5
}
gradeList[i] ← gradeList[i] + 5
IF (gradeList[i] > 100)
{
	gradeList[i] ← 100
}
5.6.5. Reflection: For Your Portfolio¶
Answer the following portfolio reflection questions as directed by your instructor. Questions are also available in this Google Doc where you may use File/Make a Copy to make your own editable copy.