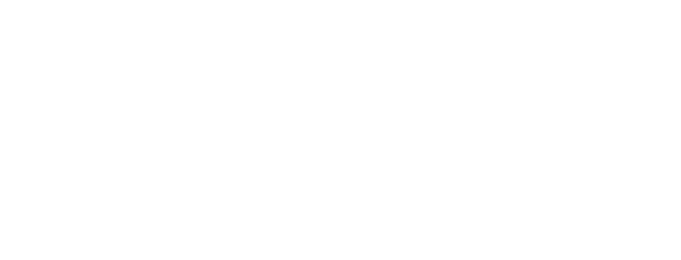
6.8. Debugging Caesar Cipher¶
Time Estimate: 45 minutes
6.8.1. Introduction and Goals¶
In this lesson, we will learn more about the types of errors you might get in a program, including tips for identifying, fixing, and preventing them.
- identify and correct errors in a program
- explain the difference between syntax errors and semantic errors
- use target vocabulary, such as syntax error, semantic error, and run-time error while fixing errors in an app, with the support of concept definitions and vocabulary notes from this lesson
6.8.2. Learning Activities¶
First, watch the video below. After watching the video, try to identify and correct the errors in the Caesar Cipher app and then answer the questions about debugging. When watching the video, look for these important terms and concepts:
- Bug - In computer programming, a bug is an error or defect that prevents the app from working the way it is supposed to.
- Debugging - The process of removing errors from computer hardware or software.
- Logic Error - A mistake in the algorithm or program that causes it to behave incorrectly or unexpectedly. Also referred to as a semantic error.
- Syntax Error - A mistake in the program where the rules of the programming language are not followed.
Activity
The activity for this lesson is to debug a version of the Caesar cipher app. There are at least five errors in this version of the app. See if you can find and correct them all! To get started, open App Inventor with the Caesar Cipher Buggy template.
Here are some hints and suggestions.
- If you see a run-time error message, read it carefully - it's trying to tell you where the bug is. A run-time error is a mistake in the program that occurs during the execution of a program. Programming languages, such as MIT App Inventor, define their own run-time errors. For example, if the run-time error complains about exceeding the length of the text, then the loop going through the text letter by letter did not stop at the end of the text.
- The bugs can be in both the encryption and decryption steps. So make sure you test the app thoroughly, with appropriate inputs. When you are testing this app, only type in lowercase letters in the plaintext textbox to encrypt, and only type in uppercase letters in the Ciphertext textbox to decrypt.
- You are may see more problems if you encrypt longer rather than shorter messages.
- If you are having trouble locating a problem, use a Notifier or use Label1 to display intermediate values of local or global variables.
- You may also compare this code to your finished Caesar Cipher app from the previous lesson.
- Use App Inventor's Do It tool to evaluate expressions and intermediate values. Here's a short video on how to use Do It.
6.8.3. Summary¶
In this lesson, you learned how to:
6.8.4. Self-Check¶
Here is a table of some of the technical terms discussed in this lesson. Hover over the terms to review the definitions.
debugging
computer bug syntax error logic/semantic error run-time error |
- True
- That's right! In fact, the term "bug" was used in an account by computer pioneer Grace Hopper regarding an error that was found to be related to a moth that was trapped in the machine.
- False
- OK, so you didn’t get it right this time. Let’s look at this as an opportunity to learn. Try reviewing this; the term "bug" was actually used in an account by computer pioneer Grace Hopper regarding an error that was found to be related to a moth that was trapped in the machine.
Q-2:
True or False: In computer programming, a bug is an error or defect that prevents the app from working the way it is supposed to.
- programmer's knowledge
- Mistakes are welcome here! Try reviewing this; the programmer's knowledge can be used to determine if statements are formulated correctly, this is not what is meant by syntax.
- compiler
- Mistakes are welcome here! Try reviewing this; the compiler runs the program statements whether or not they are formulated correctly. If there is a syntax error, the compiler will inform you that there is an error.
- programming language
- Mistakes are welcome here! Try reviewing this; the programming language is the language the statements are written in. The programming language itself does not determine if the statements are formulated correctly. The programming language's syntax does this.
- set of rules
- That's right! Programming languages all have syntax, a set of rules, that must be followed when writing code.
Q-3:
In computer programming, syntax is the __________ that determines whether statements are correctly formulated.
- semantic
- Mistakes are welcome here! Try reviewing this...Syntax errors occur when a programming language's rules for writing code are broken. The compiler can detect syntax errors and report an error message to the programmer.
- syntax
- That's right! Syntax errors occur when a programming language's rules for writing code are broken. The compiler can detect syntax errors and report an error message to the programmer.
Q-4: A ____________ error occurs when a programming language’s rules are broken. This type of error can be detected by the compiler which will provide an error message.
- Semantic
- That's right! Semantic errors occur when the programmer unintentionally writes code that follows the syntax rules of the programming language, but their code works in a different way than what the programmer had intended it to.
- Syntax
- OK, so you didn’t get it right this time. Let’s look at this as an opportunity to learn. Try reviewing this; semantic errors occur when the programmer unintentionally writes code that follows the syntax rules of the programming language, but their code works in a different way than what the programmer had intended it to.
Q-5: A ____________ error occurs when a programmer inadvertently puts code that is syntactically correct, but does not do what the programmer intended it to do.
- True
- We’re in the learning zone today. Mistakes are our friends!
- False
- That's right! Semantic errors cannot be detected by the compiler. Only the programmer knows what it wants the program to do.
Q-6:
True or False: Semantic errors can be detected by the compiler.
- The PaintPot ButtonMinus.Click event adding 1 to dotsize.
- True. This would be syntactically correct, however based on the documentation (the name of the button) it would not do what the programmer had intended it to, which would be to decrease the dotsize by 1 when ButtonMinus is clicked.
- Coding duplicate Canvas1.Touched event handlers in PaintPot.
- We’re in the learning zone today. Mistakes are our friends!
- Attempting to set PaintPot's Canvas1.PaintColor to red using a text block.
- We’re in the learning zone today. Mistakes are our friends!
- The PaintPot ButtonRed.Click setting the Canvas1.PaintColor to blue.
- True. This would be correct syntactically, however, based on the documentation (the name of the button) it would not do what the programmer had intended it to, which would be to set the paint color to red when the ButtonRed is clicked.
Q-7:
Which of the following are examples of semantic errors in App Inventor? Check all that apply.
- Rebuild the app and then retest the app
- Here are some strategies to figure this out. The programmer should not rebuild their entire app just because there is a bug in it. They should take their time to examine the code and form a hypothesis about what the bug may be. Then they should design and implement an experiment, and then debug and retest their app.
- Form a hypothesis about what might be wrong, design an experiment to test their hypothesis, perform the experiment, debug, and then retest the app.
- That's right! When debugging a program, a programmer should form a hypothesis about what the bug might be, then design and implement an experiment to test their hypothesis. If their hypothesis was correct, then the programmer should debug the app and retest. If their hypothesis was wrong, the programmer should form a new hypothesis
- Form a hypothesis and then retest the app.
- Here are some strategies to figure this out. He/she should form a hypothesis about what could possible be wrong with the code, however, just forming a hypothesis and then retesting the app is not enough.
- Immediately start changing code and retest the app.
- Here are some strategies to figure this out. It is not recommended that he/she start changing code without first thinking about, and forming an hypothesis, about what could possible be wrong. The programmer should take their time, form a hypothesis, design and implement an experiment, and then debug and retest theirapp.
Q-8: Your classmate discovers that their LightsOff app has a bug in it. What should he/she do to debug their app?
6.8.5. Reflection: For Your Portfolio¶
Answer the following portfolio reflection questions as directed by your instructor. Questions are also available in this Google Doc where you may use File/Make a Copy to make your own editable copy.