9.9.4. Trio Student Solution 3¶
The following is a free response question from 2014. It was question 4 on the exam. You can see all the free response questions from past exams at https://apstudents.collegeboard.org/courses/ap-computer-science-a/free-response-questions-by-year.
Question 4. The menu at a lunch counter includes a variety of sandwiches, salads, and drinks. The menu also allows a
customer to create a “trio,” which consists of three menu items: a sandwich, a salad, and a drink. The price
of the trio is the sum of the two highest-priced menu items in the trio; one item with the lowest price is free.
Each menu item has a name and a price. The four types of menu items are represented by the four classes
Sandwich
, Salad
, Drink
, and Trio
. All four classes implement the following MenuItem
interface.
public interface MenuItem
{
/**
* @return the name of the menu item
*/
String getName();
/**
* @return the price of the menu item
*/
double getPrice();
}
The following diagram shows the relationship between the MenuItem
interface and the Sandwich
, Salad
, Drink
, and Trio
classes.
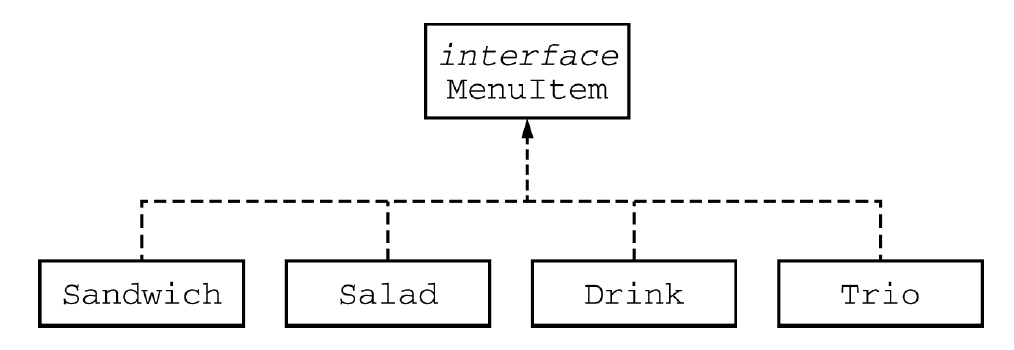
Figure 1: UML class diagram showing MenuItem is implemented by Sandwich, Salad, Drink, and Trio.¶
For example, assume that the menu includes the following items. The objects listed under each heading are instances of the class indicated by the heading.
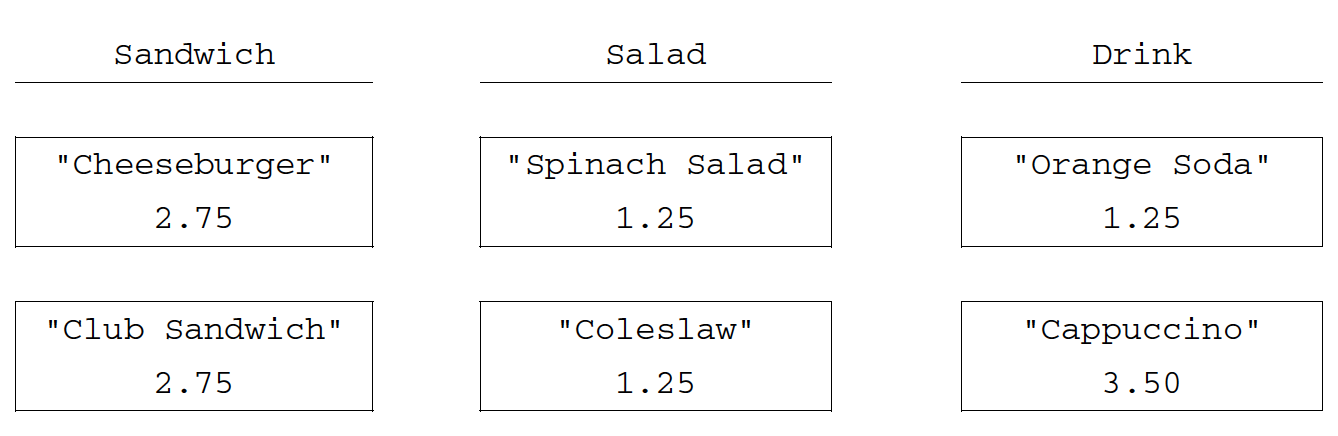
Figure 2: Example objects¶
The menu allows customers to create Trio menu items, each of which includes a sandwich, a salad, and a
drink. The name of the Trio consists of the names of the sandwich, salad, and drink, in that order, each
separated by “/” and followed by a space and then “Trio”. The price of the Trio is the sum of the two
highest-priced items in the Trio; one item with the lowest price is free.
A trio consisting of a cheeseburger, spinach salad, and an orange soda would have the name
"Cheeseburger/Spinach Salad/Orange Soda Trio"
and a price of $4.00 (the two highest prices
are $2.75 and $1.25). Similarly, a trio consisting of a club sandwich, coleslaw, and a cappuccino would have the
name "Club Sandwich/Coleslaw/Cappuccino Trio"
and a price of $6.25 (the two highest prices
are $2.75 and $3.50).
9.9.4.1. Grading Rubric¶
Below is the grading rubric for the Trio class problem.
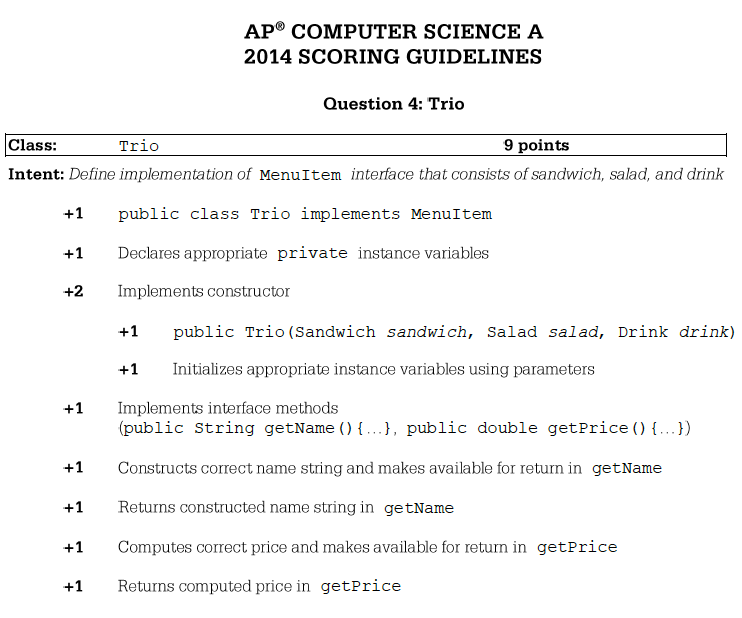
Figure 3: The grading rubric for the Trio class problem.¶
9.9.4.2. Practice Grading¶
The following is the third sample student response.
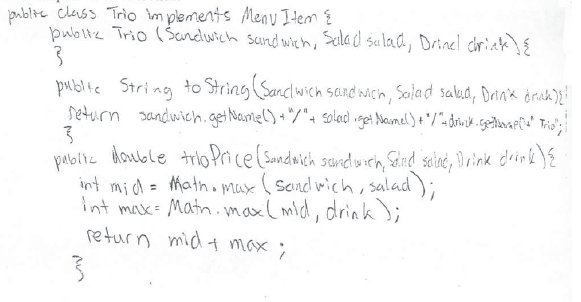
Figure 4: The start of the second sample student response to the Trio class problem.¶
Apply the grading rubric shown above as you answer the following questions.
Apply the Grading Rubric
- Yes
- This declares the class correctly as
public class Trio implements MenuItem
- No
- What do you think is wrong with the class declaration?
9-9-3-1: Should the student earn 1 point for the correct declaration of the Trio
class?
- Yes
- Do you see any instance variables declared here?
- No
- The student did not declare any instance variables.
9-9-3-2: Should the student earn 1 point for declaring the private instance variables (sandwich, salad, and drink or name and price)?
- Yes
- This solution declares the constructor as
public Trio(Sandwich sandwich, Salad salad, Drink drink)
- No
- What do you think is wrong with the constructor declaration?
9-9-3-3: Should the student earn 1 point for declaring the the constructor correctly?
- Yes
- This solution doesn't have any instance variables declared and doesn't try to use the parameter values.
- No
- There is no attempt to set the instance variables (which haven't been declared) to the parameter values.
9-9-3-4: Should the student earn 1 point for correctly initializing the appropriate instance variables in the constructor?
- Yes
- To implement an interface the class must have both a
getName
andgetPrice
method. - No
- This class is missing both the interface methods.
9-9-3-5: Should the student earn 1 point for correctly declaring the methods in the MenuItem
interface (getName and getPrice)?
- Yes
- While the
toString
method exists and correctly creates the name string, it is not called by agetName
method. - No
- Since there is no
getName
method this point can not be awarded.
9-9-3-6: Should the student earn 1 point for correctly constructing the string to return from getName
and making it available to be returned?
- Yes
- While the
toString
method exists and correctly creates and returns the name string, it is not called by agetName
method. - No
- Since there is no
getName
method this point can not be awarded.
9-9-3-7: Should the student earn 1 point for returning a constructed string from getName
?
- Yes
- While there is a method that calculates the price correctly, it is the wrong method.
- No
- There is no
getPrice
method so the student can not earn this point.
9-9-3-8: Should the student earn 1 point for correctly calculating the price and making it available to be returned from getPrice
?
- Yes
- While there is a method that calculates the price correctly and returns it, it is the wrong method.
- No
- There is no
getPrice
method so the student can not earn this point.
9-9-3-9: Should the student earn 1 point for returning the calculated price in getPrice
?