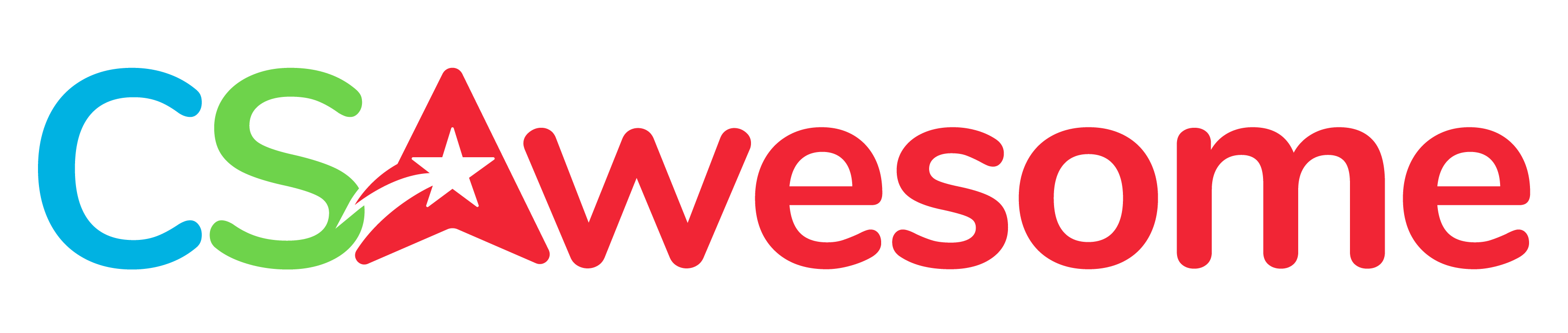
AP CSA Java Course¶
Welcome to CSAwesome! It’s time to start your journey to learn how to program with Java. A shortcut way to get to this site is to type in the url: course.csawesome.org
CSAwesome is a College Board endorsed curriculum for AP Computer Science A, an introductory college-level computer programming course in Java. If you are a teacher using this curriculum, please join the teaching CSAwesome group which will give you access to teacher resources at csawesome.org.
To make sure the site saves your answers on questions, please click on the person icon at the top to register or login to your Runestone course. As you complete lessons, click the “Mark as completed” button at the bottom. Enjoy the journey!
ATTENTION high school women of color taking AP CSA or CSP: if you identify as female and as Black, Hispanic/Latina, and/or Native American, apply to participate in Sisters Rise Up. The goal of Sisters Rise Up is to help you succeed in your AP Computer Science course and on the exam. They offer one-hour help sessions several times a week and once a month special help sessions often with guest speakers from computing. If you enroll in Sisters Rise Up and send in your AP CS exam score by the end of August, you will be sent a gift card for $100. See the flyer and apply at https://tinyurl.com/55z7tyb9.
ATTENTION high school women, genderqueer, and non-binary technologists: Apply Sept. 1st until Oct. 29th for the NCWIT Award for Aspirations in Computing to be recognized for all that you do (or want to do) in technology. Visit http://www.aspirations.org/AiCHSAward for details.
CSAwesome Units:
- Unit 1: Getting Started and Primitive Types
- Unit 2: Using Objects
- Unit 3: Boolean Expressions and If Statements
- Unit 4: Iteration (Loops)
- Unit 5: Writing Classes
- Unit 6: Arrays
- Unit 7: ArrayList
- Unit 8: 2D Arrays
- Unit 9: Inheritance
- Unit 10: Recursion
- Practice units: 11, 12, 13, 14, 15
- Unit 16: Stories
Table of Contents¶
- 1. Getting Started and Primitive Types
- 1.1. Getting Started
- 1.2. Why Programming? Why Java?
- 1.3. Variables and Data Types
- 1.4. Expressions and Assignment Statements
- 1.5. Compound Assignment Operators
- 1.6. Casting and Ranges of Values
- 1.7. Unit 1 Summary
- 1.8. Mixed Up Code Practice
- 1.9. Toggle Mixed Up or Write Code Practice
- 1.10. Coding Practice
- 1.11. Multiple Choice Exercises
- 1.12. Method Signatures (preview 2026 curriculum)
- 1.13. Calling Class Methods (preview 2026 curriculum)
- 2. Using Objects
- 2.1. Objects - Instances of Classes
- 2.2. Creating and Initializing Objects: Constructors
- 2.3. Calling Methods Without Parameters
- 2.4. Calling Methods With Parameters
- 2.5. Methods that Return Values
- 2.6. Strings
- 2.7. String Methods
- 2.8. Wrapper Classes - Integer and Double
- 2.9. Using the Math Class
- 2.10. Unit 2 Summary
- 2.11. Mixed Up Code Practice
- 2.12. Toggle Mixed Up or Write Code Practice
- 2.13. Coding Practice
- 2.14. Practice Test for Objects (2.1-2.5)
- 2.15. Multiple Choice Exercises
- 2.16. Unit 2 Free Response Question (FRQ) Practice
- 2.17. Java Swing GUIs (optional)
- 3. Boolean Expressions and If Statements
- 3.1. Boolean Expressions
- 3.2. if Statements and Control Flow
- 3.3. Two-way Selection: if-else Statements
- 3.4. Multi-Selection: else-if Statements
- 3.5. Compound Boolean Expressions
- 3.6. Equivalent Boolean Expressions (De Morgan’s Laws)
- 3.7. Comparing Objects
- 3.8. Unit 3 - Summary
- 3.9. Mixed Up Code Practice
- 3.10. Toggle Mixed Up or Write Code Practice
- 3.11. Coding Practice
- 3.12. Multiple Choice Exercises
- 3.13. Magpie Chatbot Lab
- 3.14. Unit 3 Free Response Question (FRQ) Game Practice
- 3.15. More Practice (Experiment)
- 3.16. FRQ Style Coding Practice
- 4. Iteration (Loops)
- 4.1. While Loops
- 4.2. For Loops
- 4.3. Loops and Strings
- 4.4. Nested For Loops
- 4.5. Loop Analysis
- 4.6. Unit 4 Summary
- 4.7. Group Work - Remainder Operator (%)
- 4.8. Mixed Up Code Practice
- 4.9. Toggle Mixed Up or Write Code Practice
- 4.10. Coding Practice with Loops
- 4.11. Multiple Choice Exercises
- 4.12. Free Response Questions (FRQs) for Control Structures
- 4.13. Free Response - Self Divisor A
- 4.14. Free Response - String Scramble A
- 4.15. Consumer Review Lab
- 5. Writing Classes
- 5.1. Anatomy of a Java Class
- 5.2. Writing Constructors
- 5.3. Comments and Conditions
- 5.4. Accessors / Getters
- 5.5. Mutators / Setters
- 5.6. Writing Methods
- 5.7. Static Variables and Methods
- 5.8. Scope and Access
- 5.9. this Keyword
- 5.10. Social Impacts of CS
- 5.11. Unit 5 Summary
- 5.12. Mixed Up Code Practice
- 5.13. Toggle Mixed Up or Write Code Practice
- 5.14. Multiple-Choice Exercises
- 5.15. Midterm Test
- 5.16. Free Response Question (FRQ) for Classes
- 5.17. Free Response Question - Time
- 5.18. Free Response Question - APLine
- 5.19. College Board Celebrity and Data Labs
- 5.20. Design a Class for your Community Project
- 6. Arrays
- 6.1. Array Creation and Access
- 6.1.1. Declaring and Creating an Array
- 6.1.2. Using new to Create Arrays
- 6.1.3. Initializer Lists to Create Arrays
- 6.1.4. Array length
- 6.1.5. Access and Modify Array Values
- 6.1.6. Programming Challenge : Countries Array
- 6.1.7. Design an Array of Objects for your Community
- 6.1.8. Summary
- 6.1.9. AP Practice
- 6.1.10. Arrays Game
- 6.2. Traversing Arrays with For Loops
- 6.2.1. Index Variables
- 6.2.2. For Loop to Traverse Arrays
- 6.2.3. Looping From Back to Front
- 6.2.4. Looping through Part of an Array
- 6.2.5. Common Errors When Looping Through an Array
- 6.2.6. Programming Challenge : SpellChecker
- 6.2.7. Design an Array of Objects for your Community
- 6.2.8. Summary
- 6.2.9. Arrays Game
- 6.3. Enhanced For-Loop (For-Each) for Arrays
- 6.4. Array Algorithms (FRQs)
- 6.5. Unit 6 Summary
- 6.6. Mixed Up Code Practice
- 6.7. Toggle Mixed Up or Write Code Practice
- 6.8. Code Practice with Arrays
- 6.9. Multiple-Choice Exercises
- 6.10. Practice Exam for Arrays
- 6.11. More Code Practice with Arrays
- 6.1. Array Creation and Access
- 7. ArrayList
- 7.1. Intro to ArrayLists
- 7.2. ArrayList Methods
- 7.3. Traversing
ArrayList
s with Loops - 7.4. ArrayList Algorithms
- 7.5. Searching Algorithms
- 7.6. Sorting Algorithms
- 7.7. Ethics of Data Collection and Data Privacy
- 7.8. ArrayList Summary
- 7.9. Input Files (Optional)
- 7.10. Mixed Up Code Practice
- 7.11. Toggle Mixed Up or Write Code Practice
- 7.12. Code Practice with ArrayLists
- 7.13. Multiple-Choice Exercises
- 7.14. College Board Celebrity and Data Labs
- 8. 2D Arrays
- 8.1. Two-dimensional (2D) Arrays
- 8.1.1. 2D Arrays (Day 1)
- 8.1.2. Array Storage
- 8.1.3. How Java Stores 2D Arrays
- 8.1.4. Declaring 2D Arrays
- 8.1.5. Set Value(s) in a 2D Array (Day 2)
- 8.1.6. Initializer Lists for 2D Arrays
- 8.1.7. Get a Value from a 2D Array
- 8.1.8. Programming Challenge : ASCII Art
- 8.1.9. Summary
- 8.1.10. 2D Arrays Game
- 8.2. Traversing 2D Arrays (nested loops)
- 8.2.1. Nested Loops for 2D Arrays (Day 1)
- 8.2.2. Getting the Number of Rows and Columns
- 8.2.3. Looping Through a 2D Array
- 8.2.4. AP Practice
- 8.2.5. Enhanced For-Each Loop for 2D Arrays (Day 2)
- 8.2.6. 2D Array Algorithms
- 8.2.7. 2D Array of Objects
- 8.2.8. Programming Challenge : Picture Lab
- 8.2.9. Summary
- 8.2.10. AP Practice
- 8.2.11. 2D Arrays and Loops Game
- 8.3. 2D Arrays Summary
- 8.4. Mixed Up Code Practice
- 8.5. Toggle Mixed Up or Write Code Practice
- 8.6. Code Practice with 2D Arrays
- 8.7. Free Response Questions
- 8.8. Multiple-Choice Exercises
- 8.9. College Board Picture Lab and Steganography Lab for 2D Arrays
- 8.10. More Code Practice with 2D Arrays
- 8.1. Two-dimensional (2D) Arrays
- 9. Inheritance
- 9.1. Inheritance, Superclass, Subclass
- 9.2. Inheritance and Constructors
- 9.3. Overriding Methods
- 9.4. super Keyword
- 9.5. Inheritance Hierarchies
- 9.6. Polymorphism
- 9.7. Object Superclass
- 9.8. Inheritance Summary
- 9.9. Free Response Questions
- 9.10. Mixed Up Code Practice
- 9.11. Toggle Mixed Up or Write Code Practice
- 9.12. Code Practice with Object Oriented Concepts
- 9.13. Multiple-Choice Exercises
- 9.14. College Board Celebrity Lab
- 10. Recursion
- 11. Post Test and Survey
- 12. Preparing for the Exam
- 13. Timed Multiple-Choice Exams
- 14. Mixed Up Code - Free Response Practice
- 15. Free Response Practice
- 15.1. RandomStringChooser - Part A
- 15.2. RandomStringChooser - Part B
- 15.3. StringCoder - Part A
- 15.4. StringCoder - Part B
- 15.5. StudentAnswerSheet - Part A
- 15.6. StudentAnswerSheet - Part B
- 15.7. SkyView - Part A
- 15.8. SkyView - Part B
- 15.9. Hidden Word - Write Class
- 15.10. ArrayTester - Part A
- 15.11. ArrayTester - Part B
- 15.12. NumberGroup - Part B
- 15.13. NumberGroup - Part C
- 15.14. Exercises
- 16. Stories
- 16.1. Challenges In Computing
- 16.2. Meet The Interviewees
- 16.2.1. Anaya Taylor
- 16.2.2. Bryan Hickerson
- 16.2.3. Briceida Mariscal
- 16.2.4. Carla De Lira
- 16.2.5. Camille Mbayo
- 16.2.6. Destini Deinde-Smith
- 16.2.7. Eric Espinoza
- 16.2.8. Dr. Gloria Opoku-Boateng
- 16.2.9. Dr. Juan Gilbert
- 16.2.10. Luisa Morales
- 16.2.11. Lucas Vocos
- 16.2.12. Lien Diaz
- 16.2.13. Milly Rodriguez
- 16.2.14. Dr. Nettrice Gaskins
- 17. Hidden Items
Index¶
If you see errors or bugs, please report them with this errors form. If you are a teacher who is interested in CSAwesome PDs or community, please fill out this PD interest form and join the teaching CSAwesome group which will give you access to lesson plans at csawesome.org.
(last revised 9/2024)
© Copyright 2014-2024 Barb Ericson, Univ. Michigan; 2019-2024 Beryl Hoffman, Elms College; 2023-2024 Peter Seibel, Berkeley High School. All rights reserved.
Created using Runestone.